Interactive dataframes¶
marimo makes you more productive when working with dataframes.
Display dataframes in a rich, interactive table and chart views
Transform dataframes with filters, groupbys, aggregations, and more, no code required
Select data from tables or charts and get selections back in Python as dataframes
marimo integrates with Pandas and Polars dataframes natively.
Displaying dataframes¶
marimo lets you page through, search, sort, and filter dataframes, making it extremely easy to get a feel for your data.
Display dataframes by including them in the last expression of the cell, just like any other object.
import pandas as pd
df = pd.read_json(
"https://raw.githubusercontent.com/vega/vega-datasets/master/data/cars.json"
)
df
import polars as pl
df = pl.read_json(
"https://raw.githubusercontent.com/vega/vega-datasets/master/data/cars.json"
)
df
To opt out of the rich dataframe viewer, use mo.plain
:
df = pd.read_json(
"https://raw.githubusercontent.com/vega/vega-datasets/master/data/cars.json"
)
mo.plain(df)
df = pl.read_json(
"https://raw.githubusercontent.com/vega/vega-datasets/master/data/cars.json"
)
mo.plain(df)
Transforming dataframes¶
No-code transformations¶
Use mo.ui.dataframe
to interactively
transform a dataframe with a GUI, no coding required. When you’re done, you
can copy the code that the GUI generated for you and paste it into your
notebook.
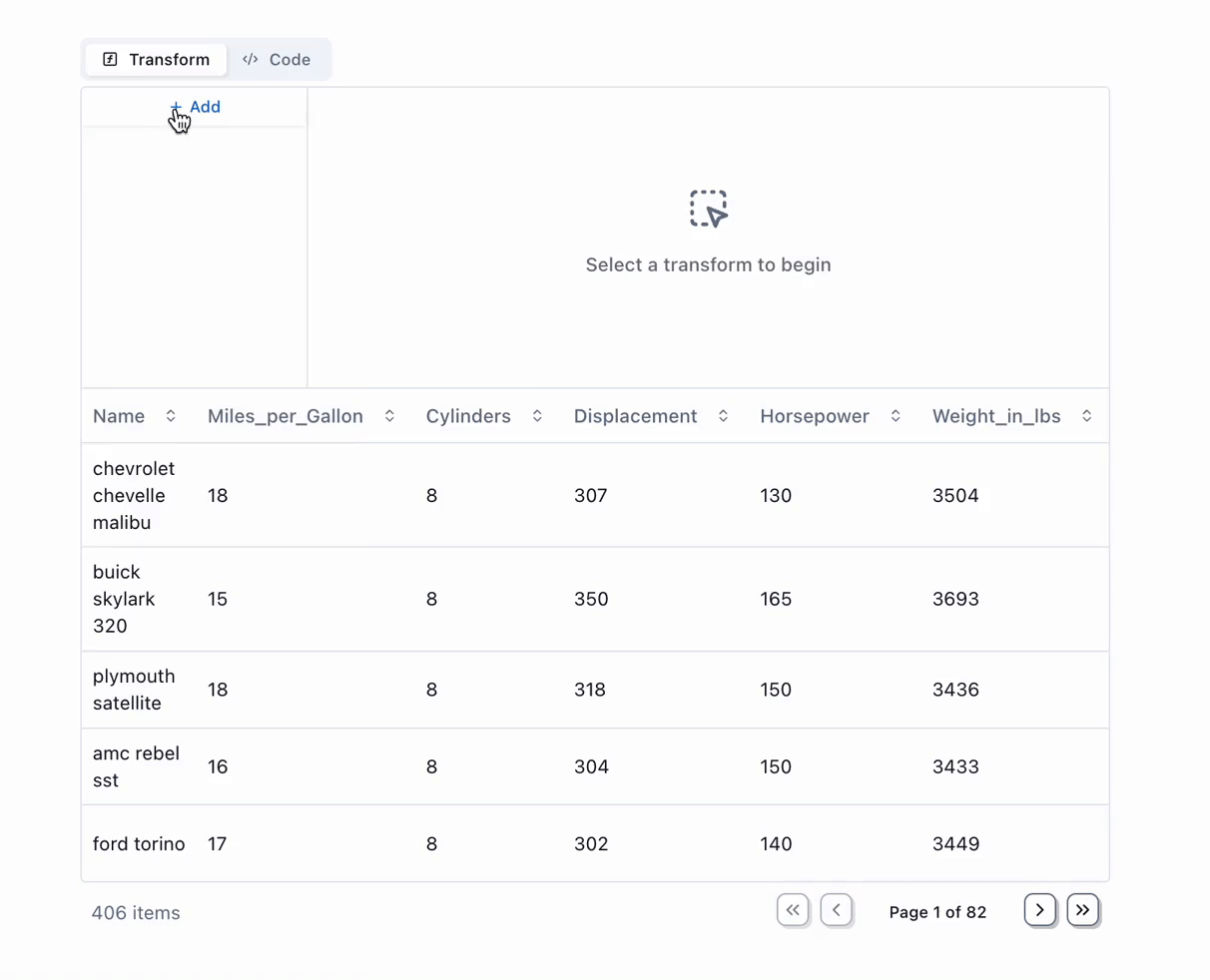
# Cell 1
import marimo as mo
import pandas as pd
df = pd.DataFrame({"person": ["Alice", "Bob", "Charlie"], "age": [20, 30, 40]})
transformed_df = mo.ui.dataframe(df)
transformed_df
# Cell 2
# transformed_df.value holds the transformed dataframe
transformed_df.value
# Cell 1
import marimo as mo
import pandas as pl
df = pl.DataFrame({"person": ["Alice", "Bob", "Charlie"], "age": [20, 30, 40]})
transformed_df = mo.ui.dataframe(df)
transformed_df
# Cell 2
# transformed_df.value holds the transformed dataframe
transformed_df.value
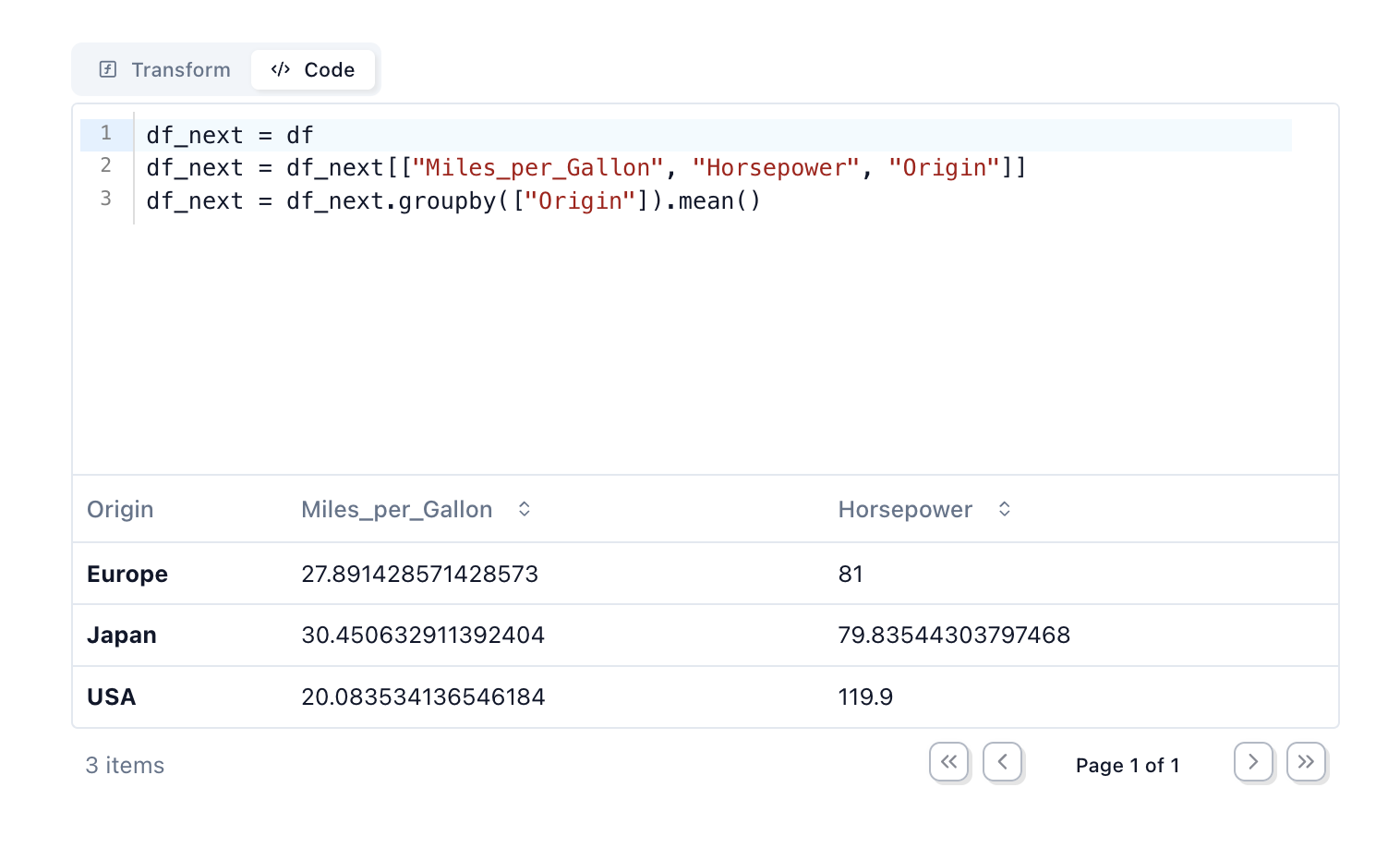
Custom filters¶
Create custom filters with marimo UI elements, like sliders and dropdowns.
# Cell 1 - create a dataframe
df = pd.DataFrame({"person": ["Alice", "Bob", "Charlie"], "age": [20, 30, 40]})
# Cell 2 - create a filter
age_filter = mo.ui.slider(start=0, stop=100, value=50, label="Max age")
age_filter
# Cell 3 - display the transformed dataframe
filtered_df = df[df["age"] < age_filter.value]
mo.ui.table(filtered_df)
import marimo as mo
import polars as pl
df = pl.DataFrame({
"name": ["Alice", "Bob", "Charlie", "David"],
"age": [25, 30, 35, 40],
"city": ["New York", "London", "Paris", "Tokyo"]
})
age_filter = mo.ui.slider.from_series(df["age"], label="Max age")
city_filter = mo.ui.dropdown.from_series(df["city"], label="City")
mo.hstack([age_filter, city_filter])
# Cell 2
filtered_df = df.filter((pl.col("age") <= age_filter.value) & (pl.col("city") == city_filter.value))
mo.ui.table(filtered_df)
Select dataframe rows¶
Display dataframes as interactive, selectable
charts using
mo.ui.altair_chart
or
mo.ui.plotly
, or as a row-selectable table with
(mo.ui.table
)(marimo.ui.table). Select points in the chart, or select a table
row, and your selection is automatically sent to Python as a subset of the original
dataframe.
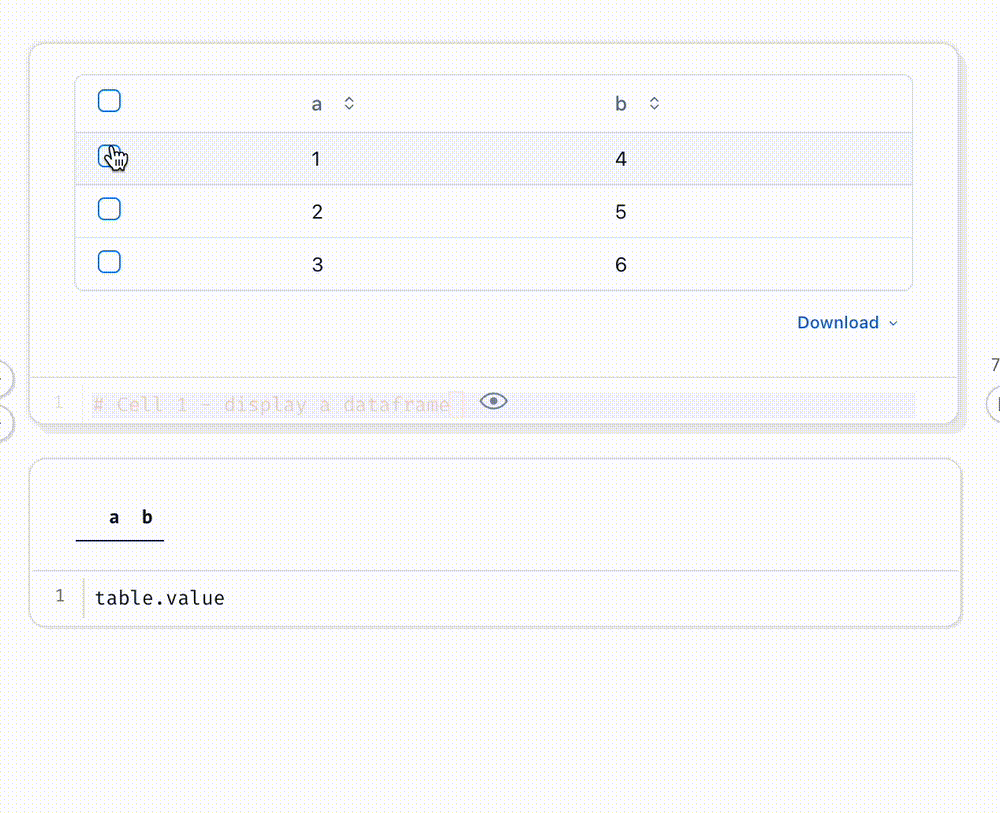
# Cell 1 - display a dataframe
import marimo as mo
import pandas as pd
df = pd.DataFrame({"a": [1, 2, 3], "b": [4, 5, 6]})
table = mo.ui.table(df, selection="multi")
table
# Cell 2 - display the selection
table.value
# Cell 1 - display a dataframe
import marimo as mo
import pandas as pl
df = pl.DataFrame({"a": [1, 2, 3], "b": [4, 5, 6]})
table = mo.ui.table(df, selection="multi")
table
# Cell 2 - display the selection
table.value
Example notebook¶
For a comprehensive example of using Polars with marimo, check out our Polars example notebook.
Run it with:
marimo edit https://raw.githubusercontent.com/marimo-team/marimo/main/examples/third_party/polars/polars_example.py